Intro
“Are you tired of constantly checking the weather forecast on your phone or computer? Look no further! In this blog post, we will show you how to create your own weather app using HTML, CSS, and JavaScript. With this app, you can quickly and easily check the weather in your area and never be caught off guard by the elements again. Best of all, we’ll provide you with the source code so you can customize it to your liking. Let’s get started!”
Recently I Started a JavaScript Projects series if you want to create these projects please check the list link given below. JavaScript Projects List.
Information About This Project
Languages | HTML, CSS, JavaScript |
Code | Available |
Preview | Take Preview |
Created by | Coding Pakistan |
Download Link | Available |
Steps to Create a Weather App Using JavaScript.
Here are the general steps to create a weather app using HTML, CSS, and JavaScript:
Step 1: First, create a basic HTML structure for your app, including the necessary tags like head
, body
, and div
.
Step 2: Use CSS to style your HTML elements. This includes designing the layout and appearance of your app, such as the background color, font, and button styles.
Step 3: Next, you’ll need to use JavaScript to connect your app to a weather API. There are many free weather APIs available that you can use to retrieve weather data for your app, such as OpenWeatherMap or WeatherAPI.
Step 4: Once you’ve connected your app to a weather API, use JavaScript to retrieve the necessary weather data (e.g., temperature, humidity, wind speed, etc.) and display it on your app.
Step 5: Consider adding additional features to your app, such as the ability to search for weather in different locations or display weather forecasts for the upcoming week.
Step 6: Finally, test your app to make sure it is functioning properly and make any necessary adjustments to the code or design.
These are the basic steps to create a weather app using HTML, CSS, and JavaScript. However, the specific implementation may vary depending on your skill level and the requirements of your project.
Video Tutorial Weather App Using JavaScript
This video is created by the Wscube Tech YouTube channel please subscribe to this channel for more videos like this one.
If you want to learn more about Weather App please watch this video till the end. I highly recommend that you do so, as I have provided detailed explanations of each line of code through written comments and demonstrated the function of each line of code.
You Might Like This
- Notes-taking app using JavaScript
- How to create an image slider using JavaScript
- Random Paragraph generator using JavaScript
- How to pass a coding interview
Build Weather App HTML, CSS & JavaScript [Source Codes]
To create a Movie App using HTML CSS & JavaScript, follow the given steps line by line:
- Create a folder. You can put any name in this folder and create the below-mentioned files inside this folder.
- Create an index.html file. The file name must be indexed and its extension .html
- Create a style.css file. The file name must be style and its extension .css
- Create a script.js file. File name must be script and its extension .js
Once you create these files, paste the given codes into the specified files. If you don’t want to do these then scroll down and download the source codes of this Password Generator by clicking on the given download button. It’s free of cost.
First, paste the following codes into your index.html file.
HTML FILE
<!DOCTYPE html> <html lang="en"> <!-- Created by Codingpakistan.com --> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> <link rel="stylesheet" href="style.css" /> </head> <body> <main> <div class="row" style="text-align: center;"> <form action=""> <input type="search" id="search" placeholder="Search by city name"> </form> </div> <div class="row" id="weather"> <!-- <div> <img src="https://openweathermap.org/img/wn/04n@2x.png" alt=""> </div> <div> <h2>32 ℃</h2> <h4> Clear </h4> </div> --> </div> </main> <script src="script.js"></script> </body> </html>
Second, paste the following codes into your style.css file.
CSS FILE
/* Created by Codingpakistan.com */ * { padding: 0; margin: 0; font-family: Verdana, Geneva, Tahoma, sans-serif; box-sizing: border-box; } main { width: 100%; height: 100vh; background-color: #3498db; display: flex; justify-content: center; align-items: center; flex-direction: column; } .row { display: flex; align-items: center; /* vertically */ justify-content: center; /* horizontally */ width: 1000px; margin: 10px; font-size: 40px; color: white; } #search { font-size: 25px; padding: 10px; border-radius: 25px; border: none; outline: none; box-shadow: 0px 0px 5px grey; }
Last, paste the following codes into your script.js file.
JavaScript FILE
// Created by codingpakistan.com const API_KEY = `3265874a2c77ae4a04bb96236a642d2f` const form = document.querySelector("form") const search = document.querySelector("#search") const weather = document.querySelector("#weather") // const API = `https://api.openweathermap.org/data/2.5/weather? // q=${city}&appid=${API_KEY}&units=metric` // const IMG_URL = `https: //openweathermap.org/img/wn/${data.weather[0].icon}@2x.png` const getWeather = async(city) => { weather.innerHTML = `<h2> Loading... <h2>` const url = `https://api.openweathermap.org/data/2.5/weather?q=${city}&appid=${API_KEY}&units=metric` const response = await fetch(url); const data = await response.json() return showWeather(data) } const showWeather = (data) => { if (data.cod == "404") { weather.innerHTML = `<h2> City Not Found <h2>` return; } weather.innerHTML = ` <div> <img src="https://openweathermap.org/img/wn/${data.weather[0].icon}@2x.png" alt=""> </div> <div> <h2>${data.main.temp} ℃</h2> <h4> ${data.weather[0].main} </h4> </div> ` } form.addEventListener( "submit", function(event) { getWeather(search.value) event.preventDefault(); } )
Weather App [Download Source Codes]
That’s all, now you’ve successfully created a Weather App using HTML CSS & JavaScript. If your code doesn’t work or you’ve faced any problems, please download the source code files from the given download button. It’s free and a zip file will be downloaded that contains the project folder with source code files.
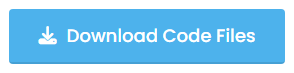