In today’s web development landscape, creating an engaging user experience is key to the success of any website. One way to achieve this is by incorporating an image slider, also known as a slideshow, into your website. An image slider is a popular and effective way to showcase multiple images or content in a single space.
In this blog post, we will walk you through how to create an image slider using HTML, CSS, and JavaScript. You don’t need to be an expert in any of these languages to follow along, but having a basic understanding will help. By the end of this tutorial, you will have a fully functional image slider that you can customize and use on your own website.
So, let’s dive in and get started with creating an image slider using HTML, CSS, and JavaScript. If you are interested in How to create a random paragraph generator please check this article first.
Steps To Create an Images Slider Using HTML, CSS & JavaScript
Step 1: Create an HTML container element:
Start by creating a container element for your image slider. This can be any HTML element, such as a div or a section, with a class or ID to identify it.
Step 2: Add images to the container:
Within the container, add the images you want to display in your image slider. You can use the HTML img element and specify the source (src) attribute to link to the image file.
Step 3: Style the container and images:
Use CSS to style the container and images. Set the container to a specific width and height, and position the images within the container using CSS positioning properties.
Step 4: Create navigation buttons:
Add navigation buttons to your image slider to allow users to move between images. You can use HTML buttons or links, and style them with CSS to match your design.
Step 5: Add JavaScript functionality:
Finally, use JavaScript to add functionality to your image slider. This can include changing the display of images when a navigation button is clicked, adding autoplay functionality, and more.
Video Tutorial Image Slider Using JavaScript
This video is created by the Wscube Tech YouTube channel please subscribe to this channel for more videos like this one.
I hope you enjoyed the Image slider demo and were able to watch the entire video tutorial. If you haven’t watched it yet, I highly recommend that you do so, as I have provided detailed explanations of each line of code through written comments and demonstrated the function of each line of code.
You Might Like This
- Age calculator using javascript
- Top 20 games using HTML, CSS and JavaScript
- Random Color generator using Javascript
- JavaScript Projects
Image Slider HTML, CSS & JavaScript [Source Codes]
To create an image slider using HTML CSS & JavaScript, follow the given steps line by line:
- Create a folder. You can put any name of this folder and create the below-mentioned files inside this folder.
- Create a index.html file. File name must be index and its extension .html
- Create a style.css file. File name must be style and its extension .css
- Create a script.js file. File name must be script and its extension .js
Once you create these files, paste the given codes into the specified files. If you don’t want to do these then scroll down and download the source codes of this Password Generator by clicking on the given download button. It’s free of cost.
First, paste the following codes into your index.html file.
HTML FILE
<!DOCTYPE html> <!-- Created by Coding Pakistan --> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <link rel="stylesheet" href="style.css"> </head> <body> <main> <img src="https://picsum.photos/id/236/1000/500" class="slide" alt=""> <img src="https://picsum.photos/id/237/1000/500" class="slide" alt=""> <img src="https://picsum.photos/id/238/1000/500" class="slide" alt=""> <img src="https://picsum.photos/id/239/1000/500" class="slide" alt=""> </main> <div class="nav"> <button onclick="goPrev()"> Prev </button> <button onclick="goNext()"> Next </button> </div> <script src="script.js"></script> </body> </html>
Second, paste the following codes into your style.css file.
CSS FILE
/* Created by Coding Pakistan */ * { padding: 0; margin: 0; box-sizing: border-box; } main { width: 60%; height: 500px; margin: auto; margin-top: 100px; box-shadow: 0px 0px 3px grey; position: relative; overflow: hidden; } .slide { width: 100%; height: 100%; transition: 1s; position: absolute; } .nav { text-align: center; margin-top: 15px; } .nav button { font-size: 25px; padding: 5px; }
Last, paste the following codes into your script.js file.
JavaScript FILE
// Created by Codingpakistan.com const slides = document.querySelectorAll(".slide") var counter = 0; // console.log(slides) slides.forEach( (slide, index) => { slide.style.top = `${index * 100}%` } ) const goPrev = () => { counter-- slideImage() } const goNext = () => { counter++ slideImage() } const slideImage = () => { slides.forEach( (slide) => { slide.style.transform = `translateY(-${counter * 100}%)` } ) }
Image Slider [Download Source Codes]
That’s all, now you’ve successfully created an images slider in HTML CSS & JavaScript. If your code doesn’t work or you’ve faced any problems, please download the source code files from the given download button. It’s free and a zip file will be downloaded that contains the project folder with source code files.
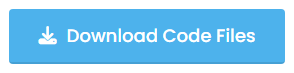