Intro
GitHub is a widely used platform for collaborative software development, and having a unique username is essential for establishing your online presence. However, finding available usernames can be a time-consuming process. This is where JavaScript comes in handy, as it can be used to automate the process of searching for available usernames on GitHub.
In this blog, we will explore how to use JavaScript to search for available usernames on GitHub and streamline the process of creating a unique and memorable username for your GitHub account. Whether you’re a developer looking to establish your online presence or simply curious about how to use JavaScript for web scraping, this blog is for you.
Recently I start a javaScript project if you are interested in JavaScript please check out this project link given below. JavaScript Projects List.
![Todo List App HTML, CSS & JavaScript [Source Codes]
To create a ToDo List app using HTML CSS & JavaScript, follow the given steps line by line:
Create a folder. You can put any name of this folder and create the below-mentioned files inside this folder.
Create a index.html file. File name must be index and its extension .html
Create a style.css file. File name must be style and its extension .css
Create a script.js file. File name must be script and its extension .js
Once you create these files, paste the given codes into the specified files. If you don’t want to do these then scroll down and download the source codes of this Password Generator by clicking on the given download button. It’s free of cost.
First, paste the following codes into your index.html file.](https://codingpakistan.com/wp-content/uploads/2023/02/github-profile-app-search-using-javascript.png)
Information About This Project
Code By | Coding Pakistan |
Project Download | link Available Below |
Languages Used | HTML, CSS, and JavaScript |
Preview | Take Preview |
Video Tutorial GitHub Profile App Using JavaScript
This video is created by the Wscube Tech YouTube channel please subscribe to this channel for more videos like this one.
If you want to learn more about GitHub Profile App please watch this video till the end. I highly recommend that you do so, as I have provided detailed explanations of each line of code through written comments and demonstrated the function of each line of code.
You Might Like This
- Todo List App Using JavaScript
- Notes App Using HTML, CSS, and JavaScript
- Random Password Generator Using JavaScript
GitHub Profile App HTML, CSS & JavaScript [Source Codes]
To create a GitHub Profile App using HTML CSS & JavaScript, follow the given steps line by line:
- Create a folder. You can put any name of this folder and create the below-mentioned files inside this folder.
- Create a index.html file. File name must be index and its extension .html
- Create a style.css file. File name must be style and its extension .css
- Create a script.js file. File name must be script and its extension .js
Once you create these files, paste the given codes into the specified files. If you don’t want to do these then scroll down and download the source codes of this Password Generator by clicking on the given download button. It’s free of cost.
First, paste the following codes into your index.html file.
HTML FILE
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <title>Github Profiles</title> <link rel="stylesheet" href="style.css" /> <script src="script.js" defer></script> </head> <body> <form id="form" onsubmit="return formSubmit()"> <input type="text" id="search" autofocus placeholder="Search a Github User Here" /> </form> <main id="main"> <!-- <div class="card"> <div> <img class="avatar" src="https://picsum.photos/200" alt="Florin Pop"> </div> <div class="user-info"> <h2>Name</h2> <p>Bio</p> <ul class="info"> <li>###<strong>Followers</strong></li> <li>##<strong>Following</strong></li> <li>###<strong>Repos</strong></li> </ul> <div id="repos"> <a class="repo" href="#" target="_blank">Repo 1</a> <a class="repo" href="#" target="_blank">Repo 2</a> <a class="repo" href="#" target="_blank">Repo 3</a> </div> </div> </div> --> </main> </body> </html>
Second, paste the following codes into your style.css file.
CSS FILE
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@200;400;600&display=swap"); * { box-sizing: border-box; padding: 0; margin: 0; } body { background-color: #2a2a72; background-image: linear-gradient(315deg, #2a2a72 0%, #2e2a68 74%); display: flex; flex-direction: column; align-items: center; justify-content: center; font-family: "Poppins", sans-serif; margin: 0; min-height: 100vh; } input { background-color: #4c2885; border-radius: 10px; border: none; box-shadow: 0 5px 10px rgba(154, 160, 185, 0.05), 0 15px 40px rgba(0, 0, 0, 0.1); color: white; font-family: inherit; font-size: 1rem; padding: 1rem; margin-bottom: 2rem; width: 400px; } input::placeholder { color: #bbb; } input:focus { outline: none; } .card { background-color: #4c2885; background-image: linear-gradient(315deg, #4c2885 0%, #4c11ac 100%); border-radius: 20px; box-shadow: 0 5px 10px rgba(154, 160, 185, 0.05), 0 15px 40px rgba(0, 0, 0, 0.1); display: flex; padding: 3rem; max-width: 800px; } .avatar { border: 10px solid #2a2a72; border-radius: 50%; height: 150px; width: 150px; } .user-info { color: #eee; margin-left: 2rem; } .user-info h2 { margin-top: 0; } .user-info ul { display: flex; justify-content: space-between; list-style-type: none; padding: 0; max-width: 400px; } .user-info ul li { display: flex; margin-right: 10px; align-items: center; } .user-info ul li strong { font-size: 0.9rem; margin-left: 0.5rem; } .repo { background-color: #2a2a72; border-radius: 5px; display: inline-block; color: white; font-size: 0.7rem; padding: 0.25rem 0.5rem; margin-right: 0.5rem; margin-bottom: 0.5rem; text-decoration: none; }
Last, paste the following codes into your script.js file.
JavaScript FILE
const APIURL = "https://api.github.com/users/"; const main = document.querySelector("#main"); const searchBox = document.querySelector("#search") const getUser = async(username) => { const response = await fetch(APIURL + username); const data = await response.json() const card = ` <div class="card"> <div> <img class="avatar" src="${data.avatar_url}" alt="Florin Pop"> </div> <div class="user-info"> <h2>${data.name}</h2> <p>${data.bio}</p> <ul class="info"> <li>${data.followers}<strong>Followers</strong></li> <li>${data.following}<strong>Following</strong></li> <li>${data.public_repos}<strong>Repos</strong></li> </ul> <div id="repos"> </div> </div> </div> main.innerHTML = card; getRepos(username) } // init call getUser("bhagirath-wscubetech") const getRepos = async(username) => { const repos = document.querySelector("#repos") const response = await fetch(APIURL + username + "/repos") const data = await response.json(); data.forEach( (item) => { const elem = document.createElement("a") elem.classList.add("repo") elem.href = item.html_url elem.innerText = item.name elem.target = "_blank" repos.appendChild(elem) } ) } const formSubmit = () => { if (searchBox.value != "") { getUser(searchBox.value); searchBox.value = "" } return false; } searchBox.addEventListener( "focusout", function() { formSubmit() } ) /** * <a class="repo" href="#" target="_blank">Repo 1</a> <a class="repo" href="#" target="_blank">Repo 2</a> <a class="repo" href="#" target="_blank">Repo 3</a> */
GitHub Profile App [Download Source Codes]
That’s all, now you’ve successfully created a GitHub Profile Search app using HTML CSS & JavaScript. If your code doesn’t work or you’ve faced any problems, please download the source code files from the given download button. It’s free and a zip file will be downloaded that contains the project folder with source code files.
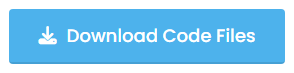